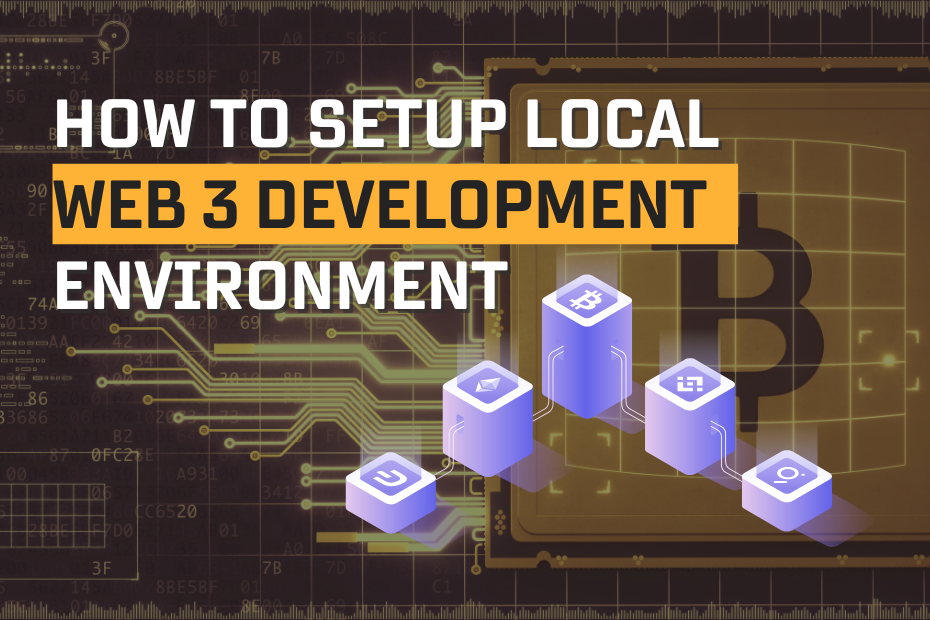
If you are looking to sharpen your web3 development skills and speed up the development speed, Setting up a local Web3 development environment is very important. A local Web3 development environment eases the overall development experience and assists in testing smart contracts without actually deploying them on the main net or test net. In this post, we are going to see different ways and means to set up your web3 development environment in a Windows environment.
While Remix is an online IDE that can help you get started in the web3 environment easily, it’s only online which means you always require an internet connection and has limited features. Moreover, you may be working with private data which you don’t want to get online. You can even run your own private Ethereum local blockchain permanently and interact with it with the tools that we are going to discuss.
Table of Contents
Best Web3 local web3 development environment
There are many tools to assist web3 development for example ganache, truffle, and hardhat. Hardhat is the most popular web3 development environment that offers a complete blockchain development experience with tools to run local blockchain and write tests and scripts for interaction with smart contracts.
For a complete and easy web3 development environment, the following tools can help you started easily:-
- Vs code
- NodeJs
- Solcjs Solidity Compiler
- EthersJS
- Git
- Ganache
- Hardhat
Setting up the essentials for Web3 development Environment
Before we are able to setup actual tools for Web3 development, we need to have some prerequisites installed and ready which are essential no matter which web3 tools we are planning to use.
1. Setup VS code for Web3 development and Solidity
VS Code is a popular IDE and has very useful extensions to make development easy and faster.
Just go to the official website and download VS Code. Now (optionally) you can install an extension called prettier that will automatically format your code once you save your code. Now press (CTRL+ ,) A settings tab will open. Now search for default formatted and change it to prettier. and set the option to format the code on save. Now install one more extension called “Solidity + Hardhat”. This will format our Solidity code.
2. Setup Node JS
Now the second step is to install NodeJS. Just visit the official website and download and install the LTS version.
We are going to see setup of two different types of web3 development environments. In the first setup we are going to see the basic setup of Ethers JS, Ganache blockchain with Solcjs compiler that provides the basic development for web3 which can be used to integrate blockchain in any nodejs project.
3. Install Git(Optional)
If you are planning to push your code to github, you can install git. To install git, you can visit this website or this one and download it. You can check the tutorials on the official website to learn how to push code on github.
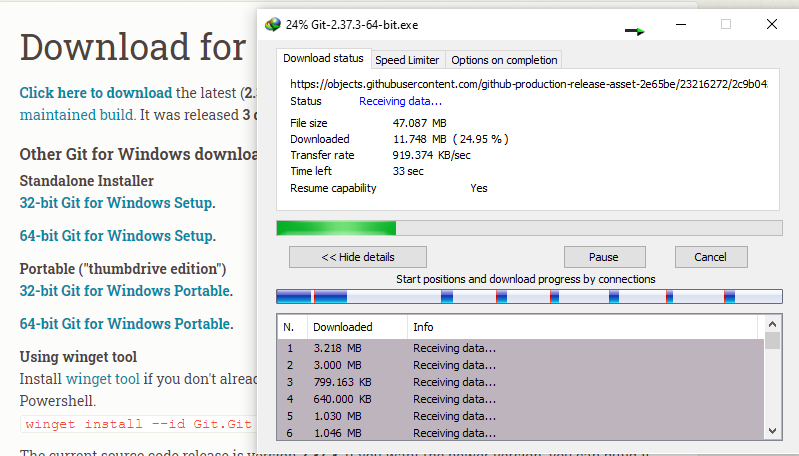
Setup EthersJs for NodeJs projects
EthersJs is a javascript library that helps to interact with blockchains. It is an alternative to Web3Js library which is another popular library for blockchain interactions. To setup a complete development environment for EtherJS we need to have a compiler as well as some mechanism to run a local blockchain.
Download and Install the Solidity compiler
Solcjs is one of the most popular solidity compilers that can be used to compile smart contracts written in Solidity. It outputs ABI as well as binary files that are used for smart contract deployment and interaction. To install a solidity compiler, open VS code and run the terminal and use npm command to install the compiler globally.
npm install -g solc
You can check if it is installed properly by running the following command.
solcjs --version
How to compile a contract in VS Code with solcjs compiler
Solcjs compiler can be used to compile a smart contract quicky in VS code with terminal. Just copy a smart contract into a new file eg:- “SimpleStorage.sol” and save it. You can use the code for testing from this GitHub Repo. (credits to free code camp)
Now we can compile a contract from code and from the command prompt as well. We are going to compile it in the terminal. use the following command in the terminal to compile our contract. We are going to get an abi file and a bin file.
solcjs --bin --abi --include-path node_modules/ --base-path . -o . SimpleStorage.sol
Here
- –bin and –abi tells that we need abi as well as binary
- –include-path will include any future dependencies from our code
- –base-path . tells look in the current directory
- -0 . tells output file in the same folder
- SimpleStorage.sol is our smart contract
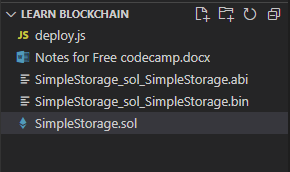
How to run a virtual blockchain with Ganache?
Ganache provides an easy mechanism to spin up a local blockchain and addresses with fake ethers so that we can make test our smart contracts and transactions. Download Ganache from the official website and install it. Once installed it provides an RPC endpoint that can be used to make connections to it.
How to install and Setup EtherJS for web3 development environment?
EtherJS library provides different methods to make calls to blockchains. You can use the following command to install the library. Just open a terminal in vs code and open the terminal and then use the command.
npm install --save ethers
and then we can use the following code in our javascript file that is going to interact with smart contract to import the library.
const { ethers } = require("ethers");
To connect to a blockchain, we need two things.
- An RPC endpoint which is shown on Ganache main screen
- A Wallet with a private key. Private keys of account can be obtained within Ganache which provides fake accounts
Now to connect to Ganache Blockchain, copy the address from ganache and create an RPC connection.
let provider = new ethers.providers.JsonRpcProvider("http://127.0.0.1:7545");
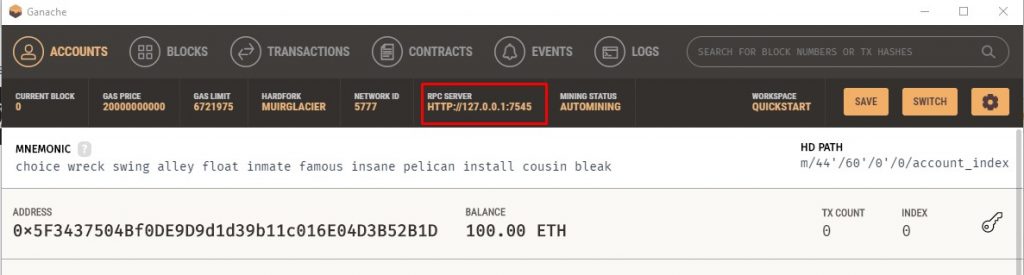
Now to create a wallet click on key icon in front of an account in Ganache and copy the private key and then create a wallet with ethersjs.
let wallet = new ethers.Wallet(
"6237e693ce483a285bfb0f8569156a84a3de40e3de36f1b43b83eb55666ae070",
provider
);
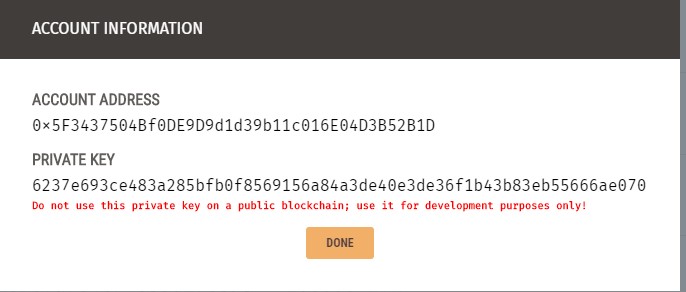
How to read compiled contracts with EtherJS?
Now for deploying the compiled contract, we need to read both the ABI and bin file. To read the file we need the fs-extra package. So, go ahead and install it with the following command.
npm install fs-extra
Now we can need to include the package in our code and then we can read both files.
const fs = require("fs-extra");
const abi = fs.readFileSync("./SimpleStorage_sol_SimpleStorage.abi", "utf8");
const binary = fs.readFileSync(
"./SimpleStorage_sol_SimpleStorage.bin",
"utf8"
);
Deploying compiled contract on Ganache with Ethersjs
After getting the compiled contract and reading it in our Javascript code with fs-extra package, we can create a contract object and then deploy it with the help of Ethersjs codefactory function.
const contractFactory = new ethers.ContractFactory(abi, binary, wallet)
console.log("Deploying, please wait...")
const contract = await contractFactory.deploy()
console.log(`Contract deployed to ${contract.address}`)
Codefactory function takes our abi, binary files and wallet private key and creates a contractFactory object which can be deployed with deploy function. The contract creation transaction can be seen in the transactions tab in Ganache as well.
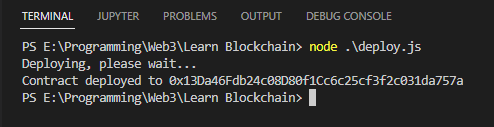
How to access functions of a deployed contract on Ganache Blockchain
To access a function for the smart contract deployed on Ganache we can use the function name with the contract address. For example to use a retrieve function on the contract address stored in the contract. We can use the contract.retrieve function.
let currentFavoriteNumber = await contract.retrieve();
console.log(`Current Favorite Number: ${currentFavoriteNumber}`);
Now to store a number on the contract, we can use the following code.
let transactionResponse = await contract.store(7);
let transactionReceipt = await transactionResponse.wait();
currentFavoriteNumber = await contract.retrieve();
console.log(`New Favorite Number: ${currentFavoriteNumber}`);
Protecting Private keys and RPC endpoints address in Web3 development
We should not use private keys and RPC endpoints in our main contract. We can use the following strategy to avoid that.
- Store the key and RPC in a separate .env file.
- include .ignore file that ignores the .env file once the project is pushed on GitHub.
- We can also encrypt the private key in .env file so that if our PC is compromised, the key remains protected.
Using .env file to store private keys
create a .env file in your project folder. Install the dotenv package with the following command.
npm install dotenv --save
Now create variables in .env file to store the private key as well as RPC endpoint
PRIVATE_KEY=12dc26e5330dba34243b7ec6509dc9ecee8dd5853f17c25ed0edcbf73378b550
RPC_URL=http://127.0.0.1:7545
Now import dotenv package in your project and use the variables as shown below:-
require("dotenv").config() //use at the top of contract
let provider = new ethers.providers.JsonRpcProvider(process.env.RPC_URL);
let wallet = new ethers.Wallet(process.env.PRIVATE_KEY, provider);
How to prevent .env files from pushing to Github
There is very simple method to prevent unwanted files pushing to Github. Just create a “.gitignore” file in the project directory and include the following file/ folder names. Now these files will not be pushed on GitHub.
node_modules
.env
How to deploy a smart contract on a testnet from VS code without Ethereum node
We can use third-party Ethereum nodes providers and connect with their nodes to deploy our contract to testnet or even main nets. A few of the most popular 3rd party Ethereum node providers include Alchemy and Infura.
How to connect to Alchemy to deploy a contract on the testnet with EtherJS
To deploy a contract on testnet with Alchemy, just go to their official website and sign up for a free account. Create a new project on a testnet for example Rinkeby testnet. Now you also need a wallet with Rinkeby funds.
Connecting with Rinkeby from Vs code
You can connect to the alchemy testnet Ethereum node with VS Code. Go to your Project on alchemy and click to get keys, now copy the HTTP address and use it as an RPC address in your Smart contract deployment code.
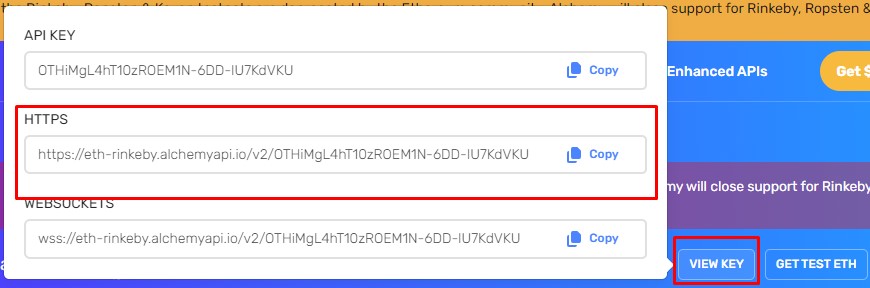
Get a private key with the balance to connect to Rinkeby
Now you can go to your metamask wallet. Change to Rinkeby testnet. Get some Rinkeby ethers. You can get Rinkeby Ethers from chainlink faucet. You can also check the tutorial to use testnets with metamask to learn about connecting to testnets and getting testnet ethers.
Now go to account settings and export your private key. Now copy the key from there and paste it in your contract deployment code.
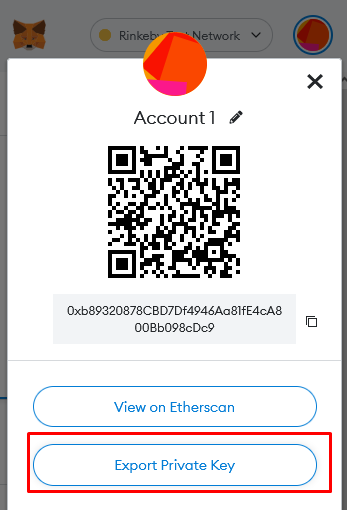
Now we have everything we need and now you can run your code. Now, this is going to take longer as we are deploying it on testnet.
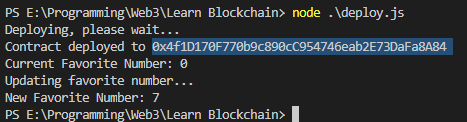
How to Setup Hardhat on Windows with VsCODE?
Hardhat is the most popular web3 development framework. Now let us see how we can create and deploy smart contracts with Hardhat. You can follow the step on Hardhat documentation. Just create a new folder and open it with VS code. Now use the following commands to initialize a project.
npm init
npm install --save-dev hardhat
Now You need to use the following command
npx hardhat
Choose the project as a basic javascript project which will provide a boilerplate to get started.
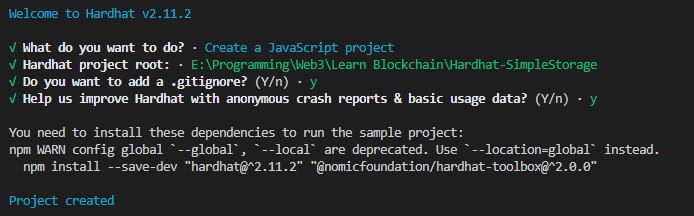
A project will be created with a number of files and folders which includes the following:-
- Contract folder with a sample contract
- scripts folder to write scripts like deploying scripts
- Node Modules
- Test folder to write some tests
- hardhat config file
- package.json
Now we need to download a toolbox that will help us to compile codes easily.
npm install --save-dev @nomicfoundation/hardhat-toolbox
Checking Hardhat tasks help
You can use the following command to check what tasks hardhat can run.
npx hardhat
It will list all tasks that can be performed currently.
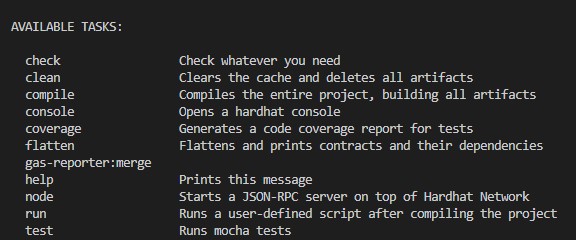
Compiling contracts in hardhat
Hardhat can be used to compile the contracts easily with a simple command. Contracts available in the contracts folder will auto-compile.
npx hardhat compile
After compiling two new folders cache and artefacts. The artefacts folder contains all information about the compiled contract. To go to the lower level interaction with the contract we can use the info in the artefacts folder.
How to compile custom smart contacts in Hard hat
First, let’s get a contract. You can use the awesome simplestorage contract from this GitHub repo and paste it into a new file simplestorage.sol. Now try to compile it. If you get a compile error, it means the compiler version and contract solidity version do not match. Simple go to hardhat.config.js and add the required solidity compiler version.
require("@nomicfoundation/hardhat-toolbox");
/** @type import('hardhat/config').HardhatUserConfig */
module.exports = {
solidity: "0.8.8",
};
Now compile the code and you will see that it’s compiling correctly.
How to deploy compiled smart contract with Hard Hat?
To deploy a smart contract with hardhat we need to write a deploy script. create a deploy.js file in the scripts folder. The deploy script looks the same as the deploy script for Ethers.js that we covered above. Here we need to import ether from hardhat.
const { ethers } = require("hardhat");
Now we will use the storagefactory function to get our contract and deploy it. We do not need ABI or binary to deploy our code. Hardhat makes it simpler as it can work only with contract names.
async function main() {
const SimpleStorageFactory = await ethers.getContractFactory("SimpleStorage");
console.log("Deploying contract...");
const simpleStorage = await SimpleStorageFactory.deploy();
await simpleStorage.deployed();
console.log(`Deployed contract to: ${simpleStorage.address}`);
main()
.then(() => process.exit(0))
.catch((error) => {
console.error(error);
process.exit(1);
});
Now we can deploy the contract on the default hardhat network with the following script Note that we do not need any RPC Url or private address to deploy on the hardhat network. Hardhat does the heavy lifting
npx hardhat run .\scripts\deploy.js

Hardhat networks
Hardhat comes with a predefined network that is used by default to interact with the contracts. If we want to add a network we can add it in the hardhat config file. To be more specific, we can specify the default network as under in harhatconfig.js file
require("@nomicfoundation/hardhat-toolbox");
/** @type import('hardhat/config').HardhatUserConfig */
module.exports = {
defaultNetwork: "hardhat",
solidity: "0.8.8",
};
we can also tell hardhat explicitly while deploying a contract to use a specific network with a network flag.
npx hardhat run .\scripts\deploy.js --network hardhat
This will tell hardhat to deploy the script to the hardhat network.
Hardhat local Host Blockchain
Hardhat can also run a temporary blockchain similar to ganache with around 10-20 wallets which we can use to interact and deploy contracts to. To run a local blockchain with the hardhat, use the following command.
npx hardhat node
It will spin up a blockchain instance and show all accounts available with test ethers already available. It will provide HTTP and Websocket RPC URL to interact with.
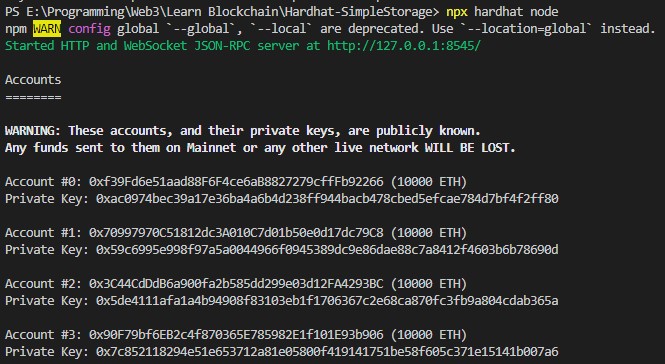
Now we can add this blockchain in our hardhat config file, so that we may be able to deploy contracts to it. Just open hardhat.config.js file and in the network section add the following network.
networks: {
hardhat: {},
localhost: {
url: "http://localhost:8545",
chainId: 31337,
},
},
We do not need to give accounts as hardhat automatically knows all accounts info.
We can now deploy our contract with the following command.
npx hardhat run .\scripts\deploy.js --network localhost

Adding other Blockchain Networks to hardhat
Blockchain networks can be simply added by including them in the harhhat.config.js file. But we need to specify the Url and private keys and chain IDs for these networks.
networks: {
hardhat: {},
goerli: {
url: GOERLI_RPC_URL,
accounts: [PRIVATE_KEY],
chainId: 5,
},
localhost: {
url: "http://localhost:8545",
chainId: 31337,
},
},
We can add the private keys and RPC URLs in the .env file. Just create a .env file and write the URLs and private keys.
PRIVATE_KEY=234523425asdfasdfa
GOERLI_RPC_URL=http://0.0.0.0:8545
Now install it with the following command
npm install dotenv --save
Now import the package in the .env file as well as the constant values.
require("dotenv").config();
const GOERLI_RPC_URL = process.env.GOERLI_RPC_URL ;
const PRIVATE_KEY = process.env.PRIVATE_KEY;
You can sign up on alchemy, create a new project and get a key. for getting a private key you can go to meta mask and in account settings, you can view the private key. Just paste it into .env file.
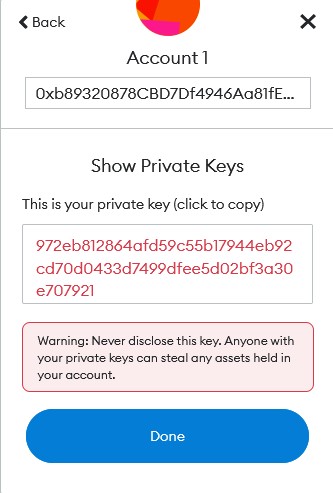
The third thing is the chain ID which can be obtained from https://chainlist.org/
Now get some testnets funds transferred to your metamask wallet from the https://goerlifaucet.com/.
Now you can deploy a contract to goerli testnet with the following command
npx hardhat run .\scripts\deploy.js --network goerli
The contact will be deployed

Now if we want to run some code after the code is deployed we can add the following line in our deploy script.
await simpleStorage.deployTransaction.wait(6);
How to Interact with smart contracts in hard hat
Interacting with contracts is very simple and the following code can be used to access the store and retrieve functions.
await simpleStorage.deployTransaction.wait(6);
const currentValue = await simpleStorage.retrieve();
console.log(`Current Value is: ${currentValue}`);
// Update the current value
const transactionResponse = await simpleStorage.store(7);
await transactionResponse.wait(1);
const updatedValue = await simpleStorage.retrieve();
console.log(`Updated Value is: ${updatedValue}`);
Now we can deploy it on harhat network with the following command
npx hardhat run .\scripts\deploy.js
Our contract will be interacted and we are going to get the values as shown

FAQ
How to use blockchain in a node js project?
Blockchain and web3 can be integrated with a node JS project with the help of a library web3.js or ether.js. These libraries provide functions to easily interact with smart contracts.
How to setup local blockchain for web3 development?
You can either run ganache which provides standalone blockchain or install a Hard Hat development environment which provides local blockchain for fast testing of smart contracts.